Equivalent to Matlab's Images in Python MatplotlibUnderstanding MaltabMatlab is a high-level programming language and interactive environment primarily used for numerical computing, visualization, and data analysis. Originally developed by MathWorks, Matlab allows users to perform matrix manipulations, implement algorithms, create user interfaces, and visualize data, among other things. It's widely used in various fields such as engineering, science, economics, and finance due to its powerful capabilities and extensive libraries. Matlab's syntax is relatively easy to learn, especially for those familiar with linear algebra concepts. It provides a range of built-in functions for tasks like linear algebra, statistics, signal processing, and optimization. Additionally, Matlab supports the creation of graphical user interfaces (GUIs) for building interactive applications. One of the key features of Matlab is its plotting and visualization capabilities. Users can create 2D and 3D plots, images, animations, and interactive presentations to analyze and communicate data effectively. Overall, Matlab is a versatile tool that enables researchers, engineers, and analysts to solve complex mathematical problems, conduct simulations, and analyze data efficiently. Let's delve a bit deeper into some theoretical aspects of Matlab. - Matrix-based Computation: At the core of Matlab is its ability to efficiently perform matrix-based computations. Matlab treats arrays as fundamental data types, and many operations are naturally extended to work with matrices. This makes it particularly well-suited for linear algebra operations, such as solving systems of linear equations, eigenvalue calculations, and singular value decompositions.
- Vectorization: Matlab encourages vectorized operations, where computations are performed on entire arrays rather than individual elements. This approach can lead to significant performance improvements compared to iterative operations, especially when dealing with large datasets.
- Functions and Scripting: Matlab supports both procedural and functional programming paradigms. Users can write scripts, which are collections of Matlab commands stored in a file, and functions, which encapsulate a sequence of operations into reusable modules. This allows for modular and organized code, promoting code reusability and readability.
- Toolboxes: Matlab's functionality can be extended through toolboxes, which are collections of specialized functions for specific domains. These toolboxes cover areas such as signal processing, image processing, control systems, optimization, and more. They provide additional functionality beyond the core Matlab environment, enabling users to tackle a wide range of problems.
- Interactive Environment: Matlab's interactive environment, including its command-line interface and integrated development environment (IDE), facilitates rapid prototyping and experimentation. Users can execute commands interactively, visualize data, and debug code in real-time, which is particularly useful for exploratory data analysis and algorithm development.
- Graphics and Visualization: Matlab offers powerful tools for creating high-quality graphics and visualizations. Users can generate various types of plots, including line plots, scatter plots, histograms, contour plots, and surface plots. Customization options allow users to tailor the appearance of plots to meet specific requirements.
- Performance Optimization: While Matlab provides a convenient environment for algorithm development, performance optimization may be necessary for computationally intensive tasks. Techniques such as preallocation, vectorization, and leveraging built-in functions can help improve the efficiency of Matlab code.
Understanding these theoretical aspects of Matlab can help users leverage its capabilities more effectively and develop efficient and robust solutions for a wide range of computational tasks. Understanding the Maltab's imagesc MethodThe `imagesc` function in Matlab is a powerful tool used for visualizing 2D data arrays as images. It stands for "image scaled," and it displays the intensity values of the input matrix as colors in a graphical representation. Here's a breakdown of how `imagesc` works and its key features: - Input Data: The primary input to `imagesc` is a 2D matrix, typically representing an image, a heat map, or any other 2D data set where each element corresponds to a pixel or a data point.
- Color Mapping: `imagesc` maps the intensity values in the input matrix to colors based on a colormap. By default, Matlab uses the colormap `parula`, but you can specify a different colormap using the `colormap` function.
- Automatic Scaling: `imagesc` automatically scales the intensity values of the input matrix to utilize the full range of colors available in the chosen colormap. This means that the minimum value in the matrix is mapped to the lowest color in the colormap, while the maximum value is mapped to the highest color.
- Colorbar: `imagesc` typically includes a colorbar alongside the image, which provides a visual reference for interpreting the intensity values and their corresponding colors.
- Interactive Exploration: `imagesc` is often used in interactive data exploration and analysis. Users can zoom in/out, pan across the image, and adjust the colormap dynamically to reveal patterns or details in the data.
Here's a simple example of how to use `imagesc` in Matlab: Matlab Output: 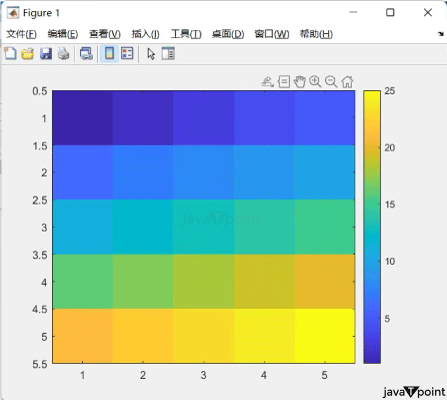 In this example, `imagesc` displays a 10x10 grid of random values as an image, with the intensity values mapped to colors according to the default `parula` colormap. The colorbar provides a scale for interpreting the colors in the image. Overall, `imagesc` is a versatile function for visualizing 2D data in Matlab, and it's commonly used in various fields such as image processing, scientific visualization, and data analysis. Understanding the Python's Matplotlib LibraryMatplotlib is a popular Python library used for creating static, interactive, and animated visualizations. It provides a wide range of plotting functionalities similar to those available in Matlab, making it a powerful tool for data visualization and exploration in Python. Here are some key features and components of Matplotlib: - Plotting Functions: Matplotlib offers a variety of plotting functions to create different types of plots, including line plots, scatter plots, bar plots, histogram plots, contour plots, surface plots, and more. These functions allow users to visualize data in various formats and representations.
- Object-Oriented Interface: Matplotlib can be used through a procedural interface, similar to Matlab's plotting functions, but it also provides an object-oriented interface that gives users more control and flexibility over their plots. With the object-oriented approach, users can create and manipulate plot objects directly, allowing for more complex and customized visualizations.
- Multiple Backends: Matplotlib supports multiple backends for rendering plots, including different graphical toolkits such as Tkinter, GTK, Qt, and web-based backends like SVG and HTML5 Canvas. This flexibility allows users to generate plots in different formats and environments, including standalone applications, web applications, and interactive notebooks like Jupyter.
- Customization and Styling: Matplotlib allows users to customize almost every aspect of their plots, including colors, linestyles, markers, fonts, labels, axes, grids, and more. Users can also apply predefined styles or create their own stylesheets to achieve consistent and visually appealing plots.
- Integration with NumPy and Pandas: Matplotlib seamlessly integrates with NumPy and Pandas, making it easy to plot data stored in NumPy arrays, Pandas DataFrames, and Series objects. This integration simplifies the process of data manipulation, analysis, and visualization within the Python ecosystem.
- Support for LaTeX: Matplotlib supports LaTeX for mathematical expressions and text rendering, allowing users to include complex mathematical notation and symbols in their plots with ease.
- Community and Ecosystem: Matplotlib has a large and active community of users and developers who contribute to its development, documentation, and support. It is widely used in various domains, including scientific research, data analysis, engineering, finance, and education.
Overall, Matplotlib is a versatile and powerful library for creating high-quality visualizations in Python, and it serves as a fundamental building block for many other visualization libraries and tools in the Python ecosystem. How Matlab's imagesc is Equivalent to Python Matplotlib?In Matplotlib, the equivalent function to Matlab's `imagesc` is `plt.imshow()`. Here's a theoretical breakdown of how `plt.imshow()` works and its key features: - Input Data: Like `imagesc`, `plt.imshow()` takes a 2D array of data as its primary input. This array represents the image or data matrix to be visualized.
- Colormap: Similar to Matlab, Matplotlib uses colormaps to map intensity values to colors. By default, Matplotlib uses the 'viridis' colormap, but you can specify any other colormap provided by Matplotlib or create custom colormaps.
- Automatic Scaling: `plt.imshow()` automatically scales the intensity values of the input data to utilize the full range of colors in the chosen colormap. This means that the minimum value in the data array is mapped to the lowest color in the colormap, while the maximum value is mapped to the highest color.
- Colorbar: Just like `imagesc`, `plt.imshow()` can include a colorbar alongside the image to provide a visual reference for interpreting the intensity values and their corresponding colors.
- Aspect Ratio: By default, `plt.imshow()` preserves the aspect ratio of the input data, ensuring that pixels are square. However, you can adjust the aspect ratio using parameters such as `aspect` and `extent`.
- Interpolation: `plt.imshow()` supports various interpolation methods for displaying the image, such as 'nearest', 'bilinear', 'bicubic', and more. Interpolation affects the smoothness of the displayed image and can be adjusted based on visualization requirements.
- Axes and Ticks: `plt.imshow()` can automatically create axes and ticks to provide spatial reference for the image. You can customize the appearance of axes, ticks, labels, and gridlines as needed.
Here's a simple example of how to use `plt.imshow()` in Matplotlib: Python Output: 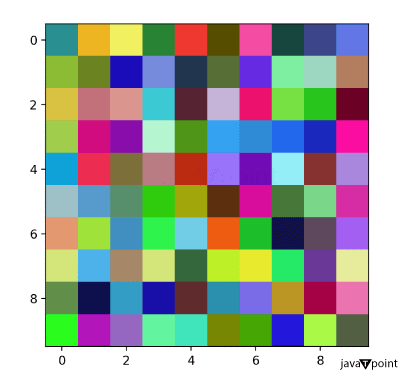 - In this example, `plt.imshow()` displays a 10x10 grid of random values as an image, with the intensity values mapped to colors according to the default 'viridis' colormap. The colorbar provides a scale for interpreting the colors in the image.
- Overall, `plt.imshow()` in Matplotlib provides similar functionality to Matlab's `imagesc` for visualizing 2D data arrays as images, making it a versatile tool for data visualization in Python.
- Multiple Data Formats: `plt.imshow()` accepts various data formats, including NumPy arrays, PIL images, and masked arrays. This flexibility allows users to visualize data from different sources and formats seamlessly.
- Image Transparency: Matplotlib supports alpha transparency for images, allowing users to control the opacity of pixels in the displayed image. This feature is useful for overlaying images or creating visual effects.
Use cases of plt.imshow()`plt.imshow()` in Matplotlib is a versatile function with a wide range of use cases for visualizing 2D data. Here are some common scenarios where `plt.imshow()` is particularly useful: - Image Display: The most straightforward use case of `plt.imshow()` is to display images stored as 2D arrays. This could be grayscale images, color images (RGB or RGBA), or images with arbitrary pixel values representing various properties.
- Heatmaps and Matrix Visualization: `plt.imshow()` is commonly used to visualize matrices or 2D arrays as heatmaps. This is useful for representing data such as correlation matrices, density plots, or any other spatially distributed data.
- Scientific Data Visualization: In scientific applications, `plt.imshow()` can be used to visualize data from experiments, simulations, or measurements. This includes displaying results from imaging techniques, spectroscopy, microscopy, and more.
- Machine Learning: In machine learning, `plt.imshow()` is often used to visualize data samples, feature representations, or intermediate layers of neural networks. For example, you can visualize convolutional filters, feature maps, or activation patterns in deep learning models.
- Geospatial Data: `plt.imshow()` can be employed to display geospatial data, such as satellite images, maps, terrain elevation data, or climate datasets. By mapping intensity values to colors, it's possible to visualize geographical features, land cover, temperature variations, and more.
- Medical Imaging: In medical imaging applications, `plt.imshow()` is used to visualize various types of medical images, including MRI scans, CT scans, X-rays, and ultrasound images. It enables medical professionals and researchers to analyze and interpret anatomical and pathological features.
- Data Analysis and Exploration: `plt.imshow()` is valuable for exploratory data analysis and visualization of numerical data. It allows users to quickly assess patterns, trends, and outliers in the data, facilitating data-driven insights and decision-making.
- Educational Purposes: `plt.imshow()` is often used in educational contexts to teach concepts related to data visualization, image processing, and scientific computing. It provides a straightforward way to illustrate mathematical concepts, algorithms, and computational techniques.
These are just a few examples of the many use cases of `plt.imshow()` in Matplotlib. Its flexibility and ease of use make it a fundamental tool for visualizing 2D data across various domains and applications in Python. Compare image display in Imagesc of Matlab and plt.imshow in MatplotlibLet's compare the image display functionality provided by `imagesc` in Matlab and `plt.imshow()` in Matplotlib: 1. Colormap Handling: - Matlab (`imagesc`): Matlab offers a variety of built-in colormaps, such as 'jet', 'hot', 'cool', 'gray', etc. Users can easily specify the desired colormap or create custom colormaps.
- Matplotlib (`plt.imshow()`): Matplotlib also provides a range of colormaps, including 'viridis', 'inferno', 'plasma', 'cividis', and more. Users can select a colormap using the `cmap` parameter, which allows for seamless integration with Matlab-like colormaps.
2. Interactive Features: - Matlab (`imagesc`): Matlab's figure windows offer interactive features like zooming, panning, and data cursor for exploring the displayed image.
- Matplotlib (`plt.imshow()`): Matplotlib's interactive capabilities depend on the environment it's used in. For example, in Jupyter notebooks, users can enable interactive features using `%matplotlib widget` magic command, allowing for zooming and panning functionalities similar to Matlab.
3. Integration with Other Libraries: - Matlab (`imagesc`): While Matlab offers extensive functionalities for numerical computing and visualization, its ecosystem may not be as extensive or flexible as Python's ecosystem.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes libraries like NumPy, Pandas, Scikit-learn, and more. This allows for seamless integration with other tools and libraries for data analysis, machine learning, and scientific computing.
4. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the displayed image, such as adjusting axes, colorbars, labels, and annotations.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the displayed image, including color scaling, interpolation methods, axis properties, labels, titles, and more. Users have fine-grained control over every aspect of the plot.
- Performance and Efficiency:
- Matlab (`imagesc`): Matlab is optimized for numerical computing and often offers fast and efficient performance for displaying large datasets.
- Matplotlib (`plt.imshow()`): Matplotlib's performance can vary depending on factors such as the size of the dataset, the complexity of the visualization, and the hardware it's running on. However, Matplotlib is generally efficient for most common use cases and offers optimization techniques for improving performance when needed.
Overall, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib provide powerful tools for displaying images and visualizing 2D data. The choice between them may depend on factors such as familiarity with the respective environments, specific visualization requirements, integration with other tools, and performance considerations. Compare Heatmaps and Matrix Visualization in Imagesc of Matlab and plt.imshow in MatplotlibLet's compare the functionalities and features of creating heatmaps and visualizing matrices using `imagesc` in Matlab and `plt.imshow()` in Matplotlib: 1. Colormap Handling: - Matlab (`imagesc`): Matlab provides a wide range of built-in colormaps, and users can easily specify the desired colormap using the `colormap` function. This allows for quick and convenient selection of colormaps suited for heatmap visualization.
- Matplotlib (`plt.imshow()`): Matplotlib also offers a variety of colormaps, and users can specify the colormap using the `cmap` parameter in `plt.imshow()`. Matplotlib's colormaps are seamlessly integrated with other plotting functions, providing consistency across different types of plots.
2. Color Scaling: - Matlab (`imagesc`): `imagesc` in Matlab automatically scales the intensity values of the input data to utilize the full range of colors in the chosen colormap. This ensures that the colormap effectively represents the data distribution.
- Matplotlib (`plt.imshow()`): Similarly, `plt.imshow()` in Matplotlib automatically scales the intensity values of the input data to fit the colormap range. Users can adjust the color scaling behavior using parameters like `vmin` and `vmax` to control the color range.
3. Interpolation: - Matlab (`imagesc`): Matlab's `imagesc` function offers interpolation options for smoothing the displayed image, including nearest-neighbor, bilinear, and bicubic interpolation methods.
- Matplotlib (`plt.imshow()`): Matplotlib also supports various interpolation methods, such as 'nearest', 'bilinear', 'bicubic', and more. Users can specify the interpolation method using the `interpolation` parameter in `plt.imshow()`.
4. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the heatmap visualization, such as adjusting axes, colorbars, labels, and annotations.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the heatmap, including axis properties, labels, titles, colorbar placement, and more. Users have fine-grained control over the visual elements of the plot.
5. Integration with Other Libraries: - Matlab (`imagesc`): Matlab's ecosystem offers extensive functionalities for numerical computing, data analysis, and visualization, but it may have limitations in terms of integration with other libraries and tools outside the Matlab environment.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes libraries like NumPy, Pandas, SciPy, and more. This allows for seamless integration with other tools and libraries for data manipulation, analysis, and machine learning.
Overall, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib provide powerful tools for creating heatmaps and visualizing matrices. The choice between them may depend on factors such as familiarity with the respective environments, specific visualization requirements, integration with other tools, and personal preference. Compare Scientific Data Visualization in Imagesc of Matlab and plt.imshow in MatplotlibWhen it comes to scientific data visualization, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib are powerful tools, but they have some differences in terms of functionality and customization options. Let's compare them: 1. Colormap Handling: - Matlab (`imagesc`): Matlab provides a variety of built-in colormaps suitable for scientific data visualization, such as 'jet', 'parula', 'hot', 'cool', and more. Users can easily select the desired colormap using the `colormap` function.
- Matplotlib (`plt.imshow()`): Matplotlib offers a similar range of colormaps, including 'viridis', 'inferno', 'plasma', 'cividis', and more. Users can specify the colormap using the `cmap` parameter in `plt.imshow()`, allowing for seamless integration with Matlab-like colormaps.
2. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the visualization, such as adjusting axes, labels, annotations, and colorbar properties. Users can easily customize the appearance of the plot to meet specific scientific visualization requirements.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the plot, including axis properties, labels, titles, colorbar placement, and more. Users have fine-grained control over the visual elements of the plot, allowing for flexible customization.
3. Interpolation: - Matlab (`imagesc`): Matlab's `imagesc` function offers interpolation options for smoothing the displayed image, including nearest-neighbor, bilinear, and bicubic interpolation methods.
- Matplotlib (`plt.imshow()`): Matplotlib also supports various interpolation methods, such as 'nearest', 'bilinear', 'bicubic', and more. Users can specify the interpolation method using the `interpolation` parameter in `plt.imshow()`, allowing for smooth visualization of scientific data.
4. Performance and Efficiency: - Matlab (`imagesc`): Matlab is optimized for numerical computing and often offers fast and efficient performance for displaying scientific data.
- Matplotlib (`plt.imshow()`): Matplotlib's performance can vary depending on factors such as the size of the dataset, the complexity of the visualization, and the hardware it's running on. However, Matplotlib is generally efficient for most common use cases and offers optimization techniques for improving performance when needed.
5. Integration with Other Libraries: - Matlab (`imagesc`): Matlab's ecosystem offers extensive functionalities for scientific computing and visualization, but it may have limitations in terms of integration with other libraries and tools outside the Matlab environment.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes libraries like NumPy, Pandas, SciPy, and more. This allows for seamless integration with other tools and libraries for scientific computing, data analysis, and machine learning.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib are powerful tools for scientific data visualization. The choice between them may depend on factors such as familiarity with the respective environments, specific visualization requirements, integration with other tools, and personal preference. Compare Machine Learning in Imagesc of Matlab and plt.imshow in MatplotlibWhen comparing machine learning visualization capabilities between `imagesc` in Matlab and `plt.imshow()` in Matplotlib, it's important to note that both tools are primarily used for data visualization and may not directly integrate with machine learning algorithms. However, they can be employed for visualizing various aspects of machine learning processes and results. Here's a comparison of their capabilities in the context of machine learning: 1. Feature Visualization: - Matlab (`imagesc`): While `imagesc` primarily visualizes 2D data arrays, it can be used to visualize features extracted from machine learning models, such as feature maps from convolutional neural networks (CNNs). However, additional preprocessing may be required to convert the feature maps into 2D arrays suitable for visualization.
- Matplotlib (`plt.imshow()`): Similarly, `plt.imshow()` can be used to visualize feature maps and other intermediate representations of data in machine learning models. It offers extensive customization options for controlling the appearance of the visualized features, allowing users to tailor the visualization to their specific needs.
2. Model Evaluation: - Matlab (`imagesc`): `imagesc` can be used to visualize evaluation metrics, such as confusion matrices, accuracy scores, and loss curves, generated during the training and evaluation of machine learning models. These metrics can be represented as 2D arrays and visualized using `imagesc`.
- Matplotlib (`plt.imshow()`): Similarly, `plt.imshow()` can be employed to visualize evaluation metrics and performance measures of machine learning models. Matplotlib's customization options allow users to create informative and visually appealing plots of model evaluation results.
3. Data Analysis: - Matlab (`imagesc`): `imagesc` can be used for exploratory data analysis (EDA) tasks in machine learning, such as visualizing patterns, distributions, and correlations in the input data. It allows users to gain insights into the characteristics of the data before building machine learning models.
- Matplotlib (`plt.imshow()`): Similarly, `plt.imshow()` is suitable for visualizing data distributions, correlations, and patterns in machine learning datasets. Matplotlib's customization options enable users to create insightful plots for data analysis and preprocessing tasks.
4. Integration with Machine Learning Libraries: - Matlab (`imagesc`): Matlab provides built-in functions and toolboxes for machine learning tasks, such as classification, regression, clustering, and dimensionality reduction. While `imagesc` can be used for visualization within the Matlab environment, it may require additional steps to integrate with machine learning algorithms and libraries.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes popular machine learning libraries such as Scikit-learn, TensorFlow, PyTorch, and Keras. `plt.imshow()` can be seamlessly integrated with these libraries for visualizing data, model outputs, and evaluation metrics.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib offer capabilities for visualizing various aspects of machine learning processes, including feature visualization, model evaluation, and data analysis. The choice between them may depend on factors such as familiarity with the respective environments, integration with machine learning libraries, and specific visualization requirements. Compare Geospacial Data in Imagesc of Matlab and plt.imshow in MatplotlibWhen it comes to visualizing geospatial data, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib can be used, but they offer different levels of flexibility and integration with geospatial libraries. Here's a comparison of their capabilities: 1. Colormap Handling: - Matlab (`imagesc`): Matlab provides a variety of built-in colormaps that can be used to represent geospatial data. Users can select a suitable colormap using the `colormap` function, allowing for customization based on the nature of the data and visualization preferences.
- Matplotlib (`plt.imshow()`): Similarly, Matplotlib offers a range of colormaps that can be applied to geospatial data using `plt.imshow()`. Users can specify the desired colormap using the `cmap` parameter, providing flexibility in visualizing different aspects of the geospatial dataset.
2. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the visualization, such as adjusting axes, labels, annotations, and colorbar properties. Users can easily customize the appearance of the plot to meet specific geospatial visualization requirements.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the plot, including axis properties, labels, titles, colorbar placement, and more. Users have fine-grained control over the visual elements of the plot, allowing for flexible customization of geospatial visualizations.
3. Integration with Geospatial Libraries: - Matlab (`imagesc`): Matlab's Mapping Toolbox provides functionalities for working with geospatial data, including map projections, georeferencing, and spatial analysis. While `imagesc` can be used for visualizing geospatial data, Matlab's Mapping Toolbox offers additional features specifically designed for geospatial applications.
- Matplotlib (`plt.imshow()`): Matplotlib can be used in conjunction with geospatial libraries such as Cartopy or Basemap to create customized geospatial visualizations. These libraries provide support for map projections, geographical features, and coordinate transformations, allowing users to create sophisticated geospatial plots using `plt.imshow()`.
4. Performance and Efficiency: - Matlab (`imagesc`): Matlab is optimized for numerical computing and often offers fast and efficient performance for visualizing geospatial data.
- Matplotlib (`plt.imshow()`): Matplotlib's performance can vary depending on factors such as the size of the dataset, the complexity of the visualization, and the hardware it's running on. However, Matplotlib is generally efficient for most common geospatial visualization tasks and offers optimization techniques for improving performance when needed.
5. Data Analysis: - Matlab (`imagesc`): `imagesc` can be used for exploratory data analysis (EDA) tasks in geospatial applications, such as visualizing spatial patterns, distributions, and correlations in the data. It allows users to gain insights into the characteristics of the geospatial dataset before performing further analysis or modeling.
- Matplotlib (`plt.imshow()`): Similarly, `plt.imshow()` can be employed for data analysis and visualization in geospatial applications. Matplotlib's customization options enable users to create informative plots for exploring spatial relationships, trends, and anomalies in the data.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib can be used for visualizing geospatial data, but they offer different levels of integration with geospatial libraries and customization options. The choice between them may depend on factors such as familiarity with the respective environments, specific geospatial visualization requirements, and integration with geospatial libraries and tools. Compare Medical Imaging in Imagesc of Matlab and plt.imshow in MatplotlibWhen comparing the visualization of medical imaging data using `imagesc` in Matlab and `plt.imshow()` in Matplotlib, several factors come into play. Here's a comparison: 1. Colormap Handling: - Matlab (`imagesc`): Matlab offers various built-in colormaps suitable for medical imaging visualization, such as 'bone', 'gray', 'hot', 'jet', etc. Users can easily select the desired colormap using the `colormap` function.
- Matplotlib (`plt.imshow()`): Similarly, Matplotlib provides a range of colormaps that can be applied to medical imaging data using `plt.imshow()`. Users can specify the colormap using the `cmap` parameter, providing flexibility in visualizing different aspects of the medical images.
2. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the visualization, such as adjusting axes, labels, annotations, and colorbar properties. Users can easily customize the appearance of the plot to meet specific requirements for medical imaging visualization.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the plot, including axis properties, labels, titles, colorbar placement, and more. Users have fine-grained control over the visual elements of the plot, allowing for flexible customization of medical imaging visualizations.
3. Interpolation: - Matlab (`imagesc`): Matlab's `imagesc` function offers interpolation options for smoothing the displayed image, including nearest-neighbor, bilinear, and bicubic interpolation methods.
- Matplotlib (`plt.imshow()`): Similarly, Matplotlib supports various interpolation methods, such as 'nearest', 'bilinear', 'bicubic', and more. Users can specify the interpolation method using the `interpolation` parameter in `plt.imshow()`, allowing for smooth visualization of medical imaging data.
4. Performance and Efficiency: - Matlab (`imagesc`): Matlab is optimized for numerical computing and often offers fast and efficient performance for visualizing medical imaging data.
- Matplotlib (`plt.imshow()`): Matplotlib's performance can vary depending on factors such as the size of the dataset, the complexity of the visualization, and the hardware it's running on. However, Matplotlib is generally efficient for most common medical imaging visualization tasks and offers optimization techniques for improving performance when needed.
5. Integration with Medical Imaging Libraries: - Matlab (`imagesc`): Matlab's Image Processing Toolbox provides functionalities for working with medical imaging data, including image enhancement, segmentation, registration, and analysis. While `imagesc` can be used for visualization within the Matlab environment, Matlab's Image Processing Toolbox offers additional features specifically designed for medical imaging applications.
- Matplotlib (`plt.imshow()`): Matplotlib can be used in conjunction with other Python libraries, such as SimpleITK, ITK, or PyDICOM, for handling medical imaging data and performing advanced image processing tasks. These libraries provide support for reading, processing, and analyzing medical image data, allowing users to create sophisticated visualizations using `plt.imshow()`.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib can be used for visualizing medical imaging data, but they offer different levels of integration with medical imaging libraries and customization options. The choice between them may depend on factors such as familiarity with the respective environments, specific medical imaging visualization requirements, and integration with medical imaging libraries and tools. Compare Data Analysis and Exploration in Imagesc of Matlab and plt.imshow in MatplotlibWhen comparing data analysis and exploration capabilities between `imagesc` in Matlab and `plt.imshow()` in Matplotlib, both tools offer powerful functionalities for visualizing and analyzing 2D data. Here's a comparison: 1. Colormap Handling: - Matlab (`imagesc`): Matlab provides a variety of built-in colormaps, and users can easily select the desired colormap using the `colormap` function. This allows for customization based on the nature of the data and visualization preferences.
- Matplotlib (`plt.imshow()`): Matplotlib offers a range of colormaps that can be applied to data visualizations using `plt.imshow()`. Users can specify the colormap using the `cmap` parameter, providing flexibility in visualizing different aspects of the dataset.
2. Customization Options: - Matlab (`imagesc`): Matlab provides options for customizing various aspects of the visualization, such as adjusting axes, labels, annotations, and colorbar properties. Users can easily customize the appearance of the plot to meet specific data analysis and exploration requirements.
- Matplotlib (`plt.imshow()`): Matplotlib offers extensive customization options for controlling the appearance of the plot, including axis properties, labels, titles, colorbar placement, and more. Users have fine-grained control over the visual elements of the plot, allowing for flexible customization of data analysis and exploration visualizations.
3. Interpolation: - Matlab (`imagesc`): Matlab's `imagesc` function offers interpolation options for smoothing the displayed image, including nearest-neighbor, bilinear, and bicubic interpolation methods.
- Matplotlib (`plt.imshow()`): Similarly, Matplotlib supports various interpolation methods, such as 'nearest', 'bilinear', 'bicubic', and more. Users can specify the interpolation method using the `interpolation` parameter in `plt.imshow()`, allowing for smooth visualization of the data.
4. Performance and Efficiency: - Matlab (`imagesc`): Matlab is optimized for numerical computing and often offers fast and efficient performance for data analysis and visualization.
- Matplotlib (`plt.imshow()`): Matplotlib's performance can vary depending on factors such as the size of the dataset, the complexity of the visualization, and the hardware it's running on. However, Matplotlib is generally efficient for most common data analysis and exploration tasks and offers optimization techniques for improving performance when needed.
5. Integration with Other Libraries: - Matlab (`imagesc`): Matlab's ecosystem offers functionalities for various data analysis tasks, including statistics, machine learning, signal processing, and more. `imagesc` can be seamlessly integrated with other Matlab functions and toolboxes for comprehensive data analysis and exploration.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes libraries like NumPy, Pandas, Scikit-learn, SciPy, and more. This allows for seamless integration with other tools and libraries for data manipulation, analysis, machine learning, and scientific computing.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib offer powerful capabilities for data analysis and exploration. The choice between them may depend on factors such as familiarity with the respective environments, specific visualization requirements, integration with other tools and libraries, and personal preference. Compare Educational Usage in Imagesc of Matlab and plt.imshow in MatplotlibWhen considering educational purposes, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib can serve as effective tools for teaching concepts related to data visualization, image processing, and scientific computing. Here's a comparison of their capabilities in educational settings: 1. Ease of Use: - Matlab (`imagesc`): Matlab is known for its ease of use and intuitive interface, making it suitable for beginners and students who are new to programming or data visualization. The `imagesc` function is straightforward to use, allowing students to quickly visualize 2D data arrays and images.
- Matplotlib (`plt.imshow()`): Matplotlib is also beginner-friendly and widely used in educational contexts due to its simplicity and versatility. While it may require some familiarity with Python syntax, `plt.imshow()` offers clear documentation and examples, making it accessible to students at various skill levels.
2. Integration with Educational Materials: - Matlab (`imagesc`): Matlab provides comprehensive documentation, tutorials, and educational resources for learning data visualization and image processing using `imagesc` and other functions. These resources can be valuable for instructors and students in educational settings.
- Matplotlib (`plt.imshow()`): Similarly, Matplotlib offers extensive documentation, tutorials, and educational materials for learning data visualization with `plt.imshow()` and other plotting functions. The Python ecosystem also provides access to a wide range of educational resources, including online courses, textbooks, and community forums.
3. Customization and Experimentation: - Matlab (`imagesc`): Matlab's graphical user interface (GUI) allows students to interactively explore different visualization options and customize the appearance of plots using the built-in tools. This hands-on approach can enhance learning and experimentation.
- Matplotlib (`plt.imshow()`): Matplotlib's object-oriented interface allows for more programmatic customization and experimentation compared to Matlab's GUI. Students can learn how to customize plots using Python code, which can deepen their understanding of data visualization principles and techniques.
4. Integration with Other Tools and Libraries: - Matlab (`imagesc`): Matlab provides an integrated environment for numerical computing, data analysis, and visualization, which can streamline the learning process for students. However, it may have limitations in terms of integrating with other programming languages and tools outside the Matlab ecosystem.
- Matplotlib (`plt.imshow()`): Matplotlib is part of the larger Python ecosystem, which includes libraries like NumPy, Pandas, Scikit-learn, and more. This allows students to explore data visualization within the context of broader topics such as data analysis, machine learning, and scientific computing.
In summary, both `imagesc` in Matlab and `plt.imshow()` in Matplotlib can be valuable tools for educational purposes, offering intuitive interfaces, extensive documentation, and opportunities for customization and experimentation. The choice between them may depend on factors such as the instructor's preferences, the educational objectives, and the overall curriculum structure.
|