Regex Lookbehind in PythonRegular expressions, regularly abbreviated as regex, are a powerful device used in pc technological know-how for looking and manipulating textual content primarily based on styles. In Python, the `re` module offers a guide for operating with regular expressions. An ordinary expression is a series of characters that outline a search sample. This pattern can consist of literal characters, which includes letters or digits, in addition to unique characters that have precise meanings inside the normal expression syntax. For instance, the `.` person matches any unmarried man or woman, `*` suits zero or greater occurrences of the preceding man or woman, and `d` fits any digit. 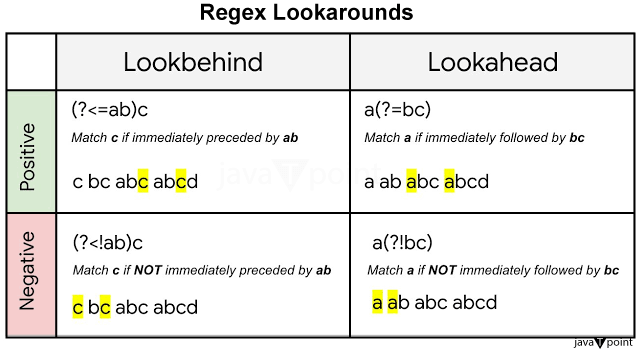 To use everyday expressions in Python, you first compile the pattern using the `re.Assemble()` function, which returns an everyday expression object. You can then use various techniques of this item to search for suits within a string, extract matching substrings, or carry out replacements. The `re.Search()` feature searches for the first incidence of the sample within the string and returns a match object if determined. The `re.Findall()` characteristic returns a listing of all non-overlapping fits of the pattern inside the string. Other beneficial functions consist of `re.Suit()` for matching patterns at the start of the string, `re.Break up()` for splitting a string based on a pattern, and `re.Sub()` for changing occurrences of a pattern with a targeted string. Regular expressions are versatile and can be used for a huge variety of duties, including validating enter, extracting information from textual content, or transforming text based on styles. However, they can also be complex and difficult to understand, for complicated patterns. It's critical to use them judiciously and to check them very well to ensure they behave as anticipated. Overall, ordinary expressions in Python provide an effective mechanism for working with text information, making an allowance for bendy and green text processing primarily based on styles. Regex lookbehind is a feature in Python's normal expression engine that lets you specify a pattern that needs to be preceded by using any other sample. Lookbehind assertions are beneficial while you need to fit a pattern best if its miles preceded via a specific series of characters, without which include those characters within the match. There are two types of lookbehind assertions in Python's regex: - Positive Lookbehind `(?<=...)`: This type of lookbehind asserts that the pattern inside the parentheses must be preceded by the pattern specified in the lookbehind. However, unlike regular matching, the characters that make up the lookbehind assertion are not included in the match.
- Negative Lookbehind `(?<!...)`: Negative lookbehind asserts that the pattern inside the parentheses must not be preceded by the pattern specified in the lookbehind.
Here's an explanation of each type with examples: Positive Lookbehind `(?<=...)`Positive lookbehind asserts that the pattern inside the parentheses must be preceded by the pattern specified in the lookbehind. However, it doesn't include the lookbehind pattern in the match. Example: Output Explanation: - `(?<=sunny\s)` is the positive lookbehind. It asserts that the match (`\w+`) must be preceded by the word "sunny" followed by a whitespace character (`\s`).
- `(\w+)` is the main pattern, matching one or more word characters.
In this example, the positive look behind ensures that only words following "sunny" are matched, without including "sunny" itself in the result. Negative Lookbehind `(?<!...)`Negative lookbehind asserts that the pattern inside the parentheses must not be preceded by the pattern specified in the lookbehind. Example: Output
['apple', 'banana', 'orange']
Explanation: - `(?<!green\s)` is the negative lookbehind. It asserts that the match (`\w+`) must not be preceded by the word "green" followed by a whitespace character (`\s`).
- `(\w+)` is the main pattern, matching one or more word characters.
In this example, the negative lookbehind ensures that only words not preceded by "green" are matched. Limitations:- Lookbehind assertions in Python support fixed-length patterns only. This means that the pattern inside the lookbehind must have a fixed length, and you cannot use quantifiers like `*` or `+`.
- Nested lookbehind assertions are not supported.
- Lookbehind assertions cannot be part of a larger alternation group.
In conclusion, regex lookbehind assertions in Python are powerful tools for matching patterns based on preceding characters or patterns. They allow you to define complex matching conditions without including the preceding characters in the match. However, it's essential to be aware of their limitations and use them judiciously in your regular expressions. Applications- Data Extraction and Parsing: Lookbehind assertions are priceless for extracting unique facts from dependent or semi-established statistics formats which include log files, CSV documents, or HTML documents. For example, think you have got a log record with timestamps within the layout YYYY-MM-DD HH:MM:SS, and also you need to extract the best time element. You can use a regex with a lookbehind assertion to suit the time preceded with the aid of the date format without capturing the date itself.
- Text Processing and Cleaning: In text processing obligations, lookbehind assertions may be employed to pick out and manipulate textual content patterns primarily based on their context. For instance, you might need to do away with all occurrences of a positive phrase simplest if it is preceded through another particular word. Lookbehind lets you precisely goal such times without altering different occurrences of the phrase.
- Tokenization and Parsing Natural Language Text: Lookbehind assertions are handy for tokenization and parsing tasks in natural language processing (NLP). They can assist in discovering specific linguistic constructs or entities based on their context within a sentence or paragraph. For example, you might use a lookbehind statement to break up a textual content into sentences based on punctuation marks while ensuring that certain abbreviations or acronyms are not wrong for sentence limitations.
- Search and Replace Operations: Lookbehind assertions beautify the precision of search and replace operations by permitting you to specify situations that should be met earlier than in shape. This functionality is mainly beneficial whilst handling complex styles or when you need to avoid unintended suits. For instance, you could use lookbehind to update all occurrences of a word most effectively if it's now not preceded by sure characters or patterns.
- Validation and Syntax Checking: Lookbehind assertions play an essential function in validating and checking the syntax of input strings in applications which includes form validation, information validation, and configuration document parsing. By incorporating lookbehind into your regex patterns, you may implement specific rules or constraints on the layout or shape of the enter data. This facilitates making certain records of integrity and consistency.
- URL and Path Matching: When working with URLs or report paths, lookbehind assertions allow you to extract or manage additives of the URL or course based on their context. For example, you can use lookbehind to extract the domain name from a URL string only if it is preceded by using the protocol part (http:// or https://) without along with the protocol itself.
- Code Refactoring and Transformation: In software improvement, regex with lookbehind can be a useful resource in code refactoring and transformation duties. For instance, you might want to update feature calls or method invocations by means of adding additional parameters handiest if certain conditions are met, consisting of the presence of specific arguments or key phrases inside the preceding code.
- Log Analysis and Monitoring: Lookbehind assertions are instrumental in log analysis and tracking systems for filtering, categorizing, or aggregating log entries primarily based on unique standards or styles inside the preceding context. This permits you to cognizance on applicable log messages or occasions while dismissing noise or beside the point facts.
Difference Between Regex Lookahead and Regex LookbehindRegex Lookahead:- Purpose: Lookahead assertions assert whether a particular sample happens beforehand (to the right) of the present-day position in the string.
- Syntax: Lookahead assertions are denoted via (?=pattern) for positive lookahead and (?!Sample) for negative lookahead.
- Usage Example: If you want to fit a word that is followed by a comma, you may use a tremendous lookahead declaration to assert the presence of a comma ahead of the word without including it within the suit: (?=w ,)w .
- Application: Useful for validating, extracting, or matching patterns that arise beforehand of the contemporary role within the string without eating characters.
Regex Lookbehind:- Purpose: Lookbehind assertions assert whether a particular pattern takes place behind (to the left) of the cutting-edge role within the string.
- Syntax: Lookbehind assertions are denoted by (?<=sample) for tremendous lookbehind and (?<!Pattern) for poor lookbehind.
- Usage Example: If you want to fit a word that is preceded via a dollar sign, you may use a nice lookbehind declaration to assert the presence of a dollar signal at the back of the phrase without which include it in the healthy: (?<=$)w .
- Application: Useful for validating, extracting, or matching patterns that arise at the back of the current role within the string without ingesting characters.
Key Differences:- Direction: Lookahead asserts appearance in advance of the present day function within the string, even as lookbehind asserts appearance in the back of the contemporary position.
- Syntax: Lookahead assertions start with (?= for high-quality lookahead and (?! For poor lookahead, whilst lookbehind assertions start with (?<= for nice lookbehind and (?<! For bad lookbehind.
- Purpose: Lookahead is used to claim styles in advance of the current position, whereas lookbehind is used to claim patterns in the back of the present day function.
- Matches: Lookahead assertions no longer devour characters, that means they simplest test for the presence or absence of a pattern without including it in the suit. Lookbehind assertions additionally do no longer eat characters but take a look at for the presence or absence of a pattern at the back of the cutting-edge role.
|